What Is Audio Focus in Android?
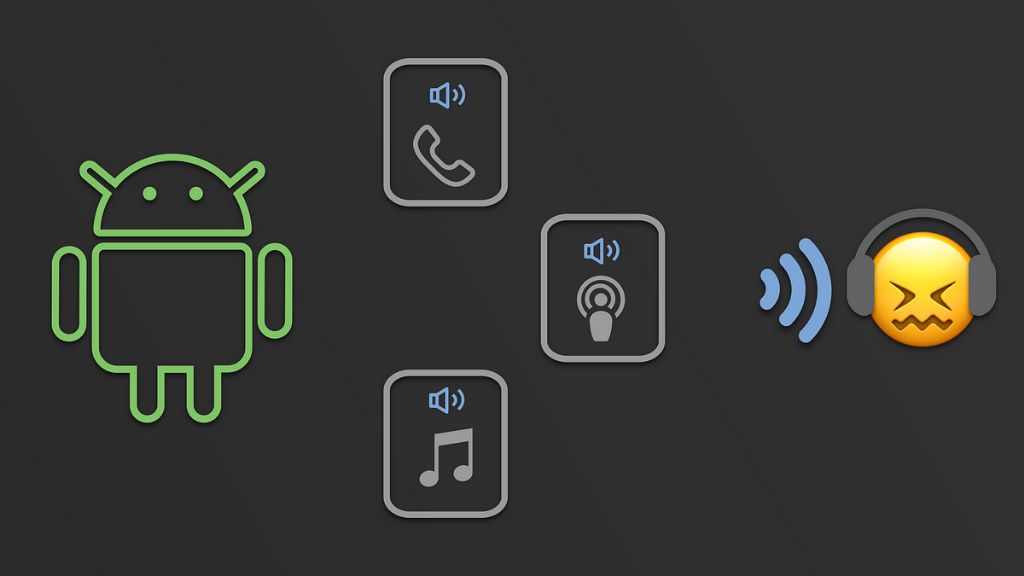
What is Audio Focus?
Audio focus in Android refers to the ability of the audio system to intelligently determine which app should have control of audio playback at a given time. It is a mechanism that allows apps to coordinate their use of audio channels and outputs (e.g. speakers, Bluetooth, etc.). This allows multiple apps to share access to audio resources while providing the best user experience.
Without audio focus, multiple apps could end up playing audio at the same time, potentially leading to overlapping, unintelligible audio. Audio focus allows the system to determine which audio source the user is most likely engaged with, and adjust volumes or pause playback accordingly. For example, if a user is playing music and then receives a phone call, the music app will automatically duck its audio volume so the call is more audible.
The audio focus system is important for avoiding audio clashes between apps and optimizing the audio experience. It ensures that audio playback aligns with user expectations and that the most relevant audio is prioritized. Apps need to properly implement audio focus APIs to integrate well with other audio apps on a device.
Why Manage Audio Focus?
Managing audio focus is important for providing a consistent user experience and avoiding awkward audio behaviors like having multiple media sources play at the same time or audio abruptly cutting out.
Without audio focus management, multiple music or media apps could end up playing audio simultaneously, creating a confusing jumble of sound for the user. Proper audio focus handling ensures that only one primary audio source plays at a time to provide a coherent experience.
Audio focus also enables smooth transitions when an interruption occurs, like an incoming call or notification. The current audio can gently fade out when another app requests focus, then resume playback afterwards. This prevents unpleasant or jarring audio cutoffs.
Overall, audio focus allows Android to coordinate audio playback across apps. By properly handling audio focus requests and callbacks, apps can operate harmoniously without conflicting audio outputs, and maintain a continuous listening experience for users.
Default Audio Focus Behavior
By default in Android, media apps have implicit audio focus. This means that media audio can play by default, unless it gets interrupted by another app requesting explicit audio focus. Apps can start playing media like music or videos without needing to explicitly acquire audio focus. However, if another app requests audio focus, it will pause the current media playback and switch audio output to the interrupting app. Once the interrupting app abandons audio focus, the previous media playback resumes automatically.
According to the Android Developer documentation, the default implicit audio focus behavior works as follows: If App A is playing music and App B requests audio focus, this will pause App A and start playing audio from App B. When App B is done with audio output and abandons focus, App A will automatically resume playback from where it left off.
This default behavior allows apps to play media freely while also allowing important interrupting apps like phone calls to take over audio output when needed. Apps don’t need to manage audio resources explicitly unless they want to deviate from the default behaviors.
Requesting Audio Focus
In order to play audio on Android, apps need to request audio focus from the AudioManager. There are a couple different ways apps can request audio focus:
Apps can request audio focus by calling requestAudioFocus()
on the AudioManager instance. This allows specifying parameters like the duration of the request (transient vs permanent) and what happens when focus is lost or gained. For example:
audioManager.requestAudioFocus(myFocusRequest, myFocusChangeListener, AudioManager.AUDIOFOCUS_GAIN);
Apps can also request focus through an AudioFocusRequest object. This allows configuring additional options like the audio attributes and focus gain hints. For example:
AudioFocusRequest focusRequest = new AudioFocusRequest.Builder(AudioManager.AUDIOFOCUS_GAIN)
.setAudioAttributes(myAttributes)
.setAcceptsDelayedFocusGain(true)
.build();
audioManager.requestAudioFocus(focusRequest);
Requests can be transient (short-lived) or permanent (long-lived). Transient requests are intended for short sounds like notifications. Permanent requests are for longer audio like music playback. Apps should request only the minimum focus needed to provide a good user experience.
Audio Focus Change Listeners
The OnAudioFocusChangeListener
interface allows an app to monitor audio focus changes so it can adjust accordingly. To register, call AudioManager.requestAudioFocus()
and pass an OnAudioFocusChangeListener
implementation as one of the parameters.
The listener provides callback methods that are invoked when audio focus changes, including:
onAudioFocusChange()
– Called when focus is gained or lost.onAudioFocusGain()
– Called when focus is gained.onAudioFocusLoss()
– Called when focus is lost.
Within these callbacks, the app should check the focus change flags and adjust audio playback accordingly. For example, if focus is lost the app may want to pause or lower the volume of audio playback. When focus returns, playback can be resumed.
Properly handling audio focus changes ensures apps play nicely together and provide a good user experience when multiple audio streams are active.
For more details, see the Android reference documentation.
Managing Audio Output
When an app gains or loses audio focus, it should properly adjust its audio output volume and behavior accordingly. This helps provide a seamless audio experience for the user.
In particular, an app that loses focus should duck its audio – lowering the volume but still playing – so the new focused app can be heard. An app that gains focus can then raise its audio volume back to normal levels.
Apps should also pause/resume audio playback appropriately based on focus changes. When an app loses focus completely, it should pause playback. When it regains focus, it can resume from where it left off.
Properly handling audio output changes ensures minimal disruption between app audio transitions. This allows users to seamlessly move between media apps without missing important audio or context.
Abandoning Audio Focus
When your app is done playing audio, it should release the audio focus so that other apps can play audio. To do this, call the AudioManager.abandonAudioFocus()
method.
This signals to the system that your app is done playing audio for now and allows other apps waiting for the audio focus to begin playing audio. This helps provide a good user experience by preventing audio from different apps from playing over each other.
Some examples of when your app should abandon focus:
- When the user pauses or stops playback
- When playback completes
- When your app moves to the background
Abandoning audio focus when appropriate allows different apps to share control of the audio output. This results in a better experience for users as they can listen to audio from multiple apps without interruption.
Audio Focus Use Cases
There are several common use cases where managing audio focus is important in Android apps:
Music/Media Playback
Media playback apps like music players, video streaming services, and podcast apps need to manage audio focus properly. They should request audio focus when playback starts and implement listeners to pause/resume based on focus changes. For example, if a VoIP call comes in, the music should duck to allow the call audio through. The music app shouldn’t just keep playing loudly over the call.
Navigation Guidance
Navigation and mapping apps need audio focus control for voice guidance prompts. The app should request audio focus when guidance prompts need to play. This ensures music or media audio will be lowered so the guidance can be heard clearly. The app should abandon focus when prompts finish.
VoIP Calling
VoIP and calling apps need to take audio focus during calls to mute/pause other media audio. When a call starts, the app should request audio focus. During the call, music or video playback should duck to allow call audio to be heard. When the call ends, the calling app should abandon focus so media playback can resume at full volume.[1]
Best Practices
When working with audio focus in Android, it’s important to follow some best practices:
Request focus with appropriate duration – When requesting audio focus using AudioManager.requestAudioFocus()
, be sure to specify an appropriate focus gain duration based on the type of audio playback. For short sounds, use AUDIOFOCUS_GAIN_TRANSIENT
. For longer playback, use AUDIOFOCUS_GAIN
or AUDIOFOCUS_GAIN_TRANSIENT_MAY_DUCK
.
Handle all focus change cases – Be sure to implement the AudioManager.OnAudioFocusChangeListener
and handle each case like AUDIOFOCUS_GAIN
, AUDIOFOCUS_LOSS
, etc. This ensures your app behaves properly when focus changes.
Abandon focus when inactive – Call AudioManager.abandonAudioFocus()
when your activity or service is no longer actively playing audio. This returns focus so other apps can resume playback.
See the AudioManager reference for more details on working with audio focus.
Audio Focus API Overview
The key classes in Android’s audio focus API include AudioManager, which manages audio focus, and AudioFocusRequest, which represents a request for audio focus. AudioManager contains constants like AUDIOFOCUS_GAIN and AUDIOFOCUS_LOSS to indicate focus changes.
To request audio focus, you call AudioManager.requestAudioFocus() and pass an AudioFocusRequest object. This method returns an int indicating the audio focus request status.
To abandon audio focus, you call AudioManager.abandonAudioFocus(). This method takes the AudioFocusRequest object you used to request focus initially.
Here’s an example usage from the Android developer documentation:
AudioFocusRequest focusRequest = new AudioFocusRequest.Builder(AudioManager.AUDIOFOCUS_GAIN) .setAudioAttributes(playbackAttributes) .setAcceptsDelayedFocusGain(true) .setOnAudioFocusChangeListener(afChangeListener) .build(); int result = audioManager.requestAudioFocus(focusRequest);
This creates an AudioFocusRequest, requests audio focus from the AudioManager, and provides a listener to be notified of focus changes.